Overview
When working with server-side ad insertion (SSAI), the Brightcove Native SDK for Android allows you to customize the ad UI elements. For more details about SSAI, see the following:
The advertisement UI is composed of two main components:
- Ad overlay (A)
- Ad media controller (B)
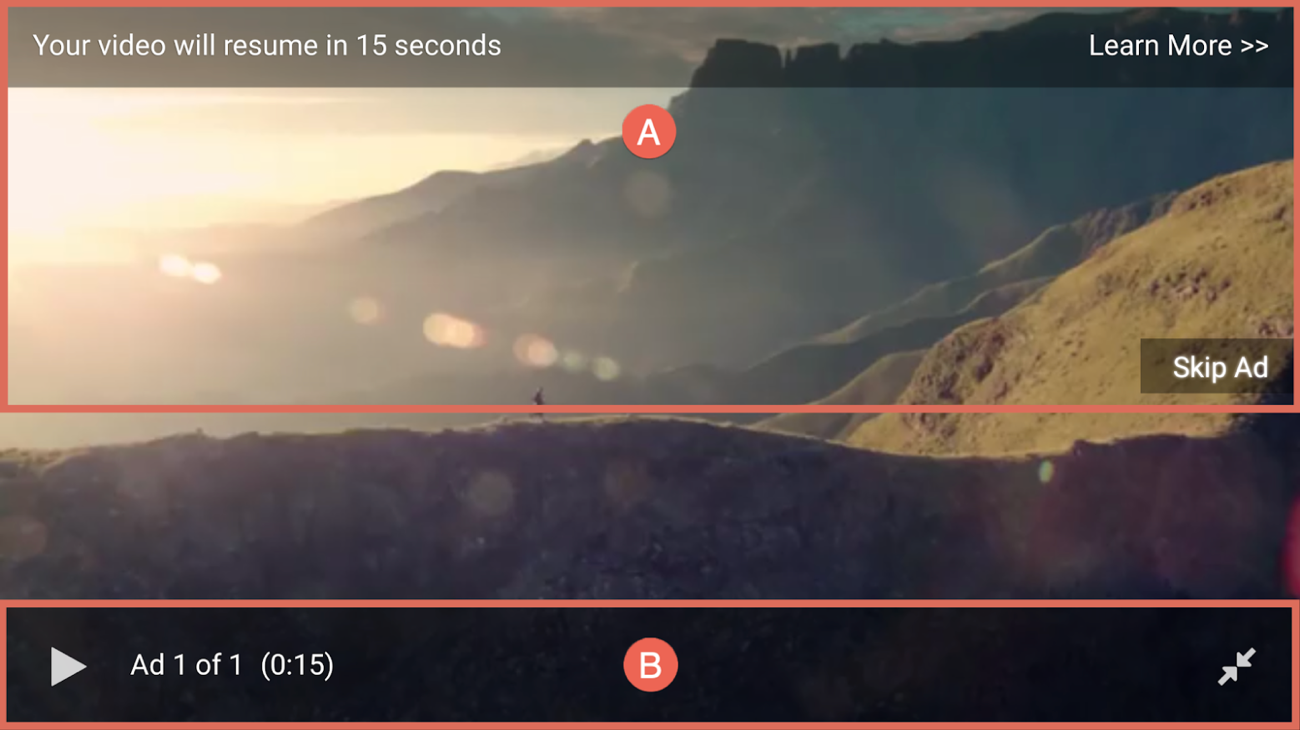
Ad overlay
The Ad Overlay is composed of these components:
- Ad Pod total duration countdown (A)
- Learn More view (B)
- Skip Ad view (C)
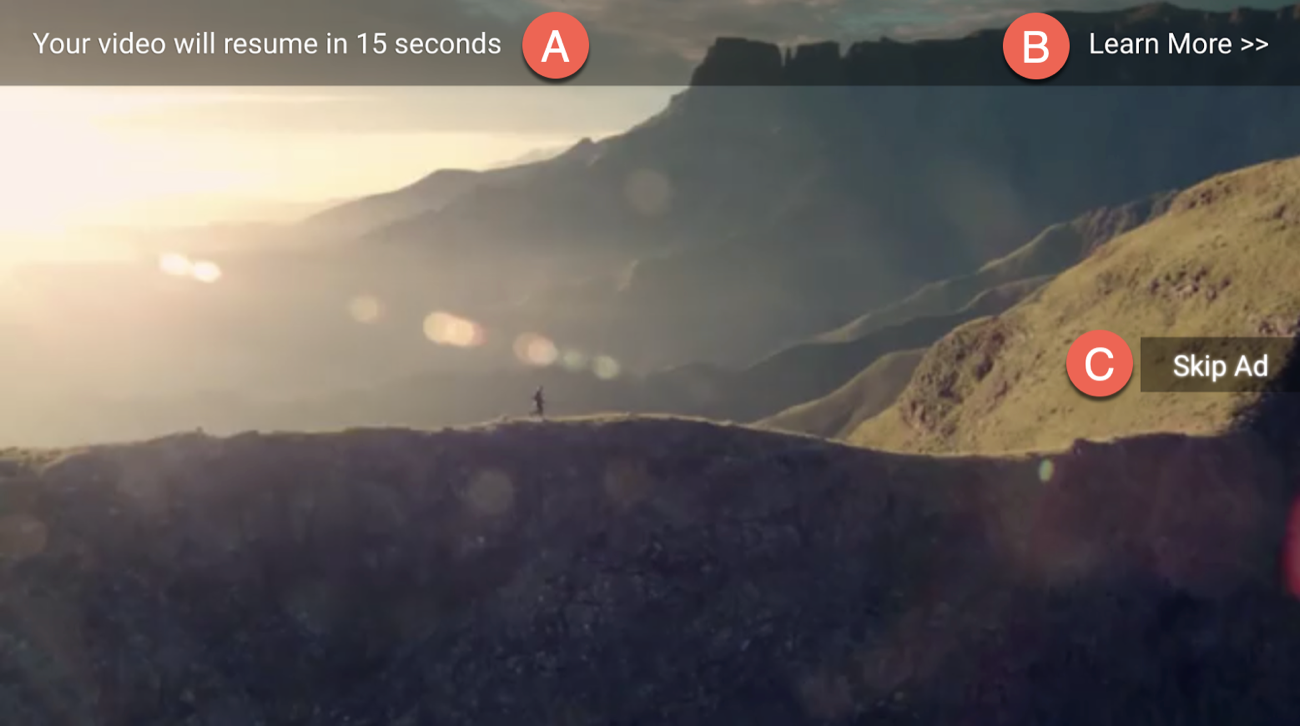
Ad media controller
The Ad media controller is composed of these components, from left to right:
- Play button (A)
- Ad number countdown (B)
- Single Ad duration countdown (C)
- Full screen button (D)

Managing ad UI components
To enable or disable ad UI components from the view, see the following:
Modifying the VAST document
The Learn More and Skip Ad views can be displayed or hidden based on the ad definition in the VAST document.
The Learn More view will appear only when the Linear Creative contains a ClickThrough
URL, as shown here:
<VAST xmlns:xsd="http://www.w3.org/2001/XMLSchema" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance">
<Ad id="blue-01-5s">
<InLine>
...
<Creatives>
<Creative id="creative-01-5s">
<Linear>
...
<VideoClicks>
<ClickThrough id="clickthrough">https://www.brightcove.com/en/</ClickThrough>
<ClickTracking id="...”>...</ClickTracking>
</VideoClicks>
</Linear>
</Creative>
</Creatives>
</InLine>
</Ad>
</VAST>
The Skip Ad view will appear only when there is a valid skipoffset
value in the Linear Creative:
<VAST xmlns:xsd="http://www.w3.org/2001/XMLSchema" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance">
<Ad id="blue-01-5s">
<InLine>
...
<Creatives>
<Creative id="creative-01-5s">
<Linear skipoffset="00:00:03">
...
</Linear>
</Creative>
</Creatives>
</InLine>
</Ad>
</VAST>
Specifying the ad configuration
When using Player Enhancements for Live SSAI, you can enable or disable components within the application_ad_configuration
of your ad configuration. The client_options
object allows you to turn ad UI components on or off.
{
"application_ad_configuration": {
"ad_configuration_description": "$YOUR_DESCRIPTION",
"ad_configuration_expected_response_type": "Vast",
"ad_configuration_headers_for_impressions": false,
"ad_configuration_strategy": "SingleAdResponse",
"ad_configuration_transforms": [],
"ad_configuration_url_format": "$YOUR_AD_SERVER",
"ad_configuration_client_sdk_enabled": true,
"client_options": {
"show_ad_break_remaining_time": true / false,
"show_ad_remaining_time": true / false,
"show_number_of_remaining_ads": true / false,
"client_only_tracking": true / false
}
},
"application_description": "$YOUR_DESCRIPTION"
}
Use the client_options object to enable/disable the following components:
Show_ad_break_remaining_time
: The Ad Pod total duration countdown- Example: Your video will resume in 15 seconds
Show_ad_remaining_time
: The single Ad duration countdown- Example: (0:15)
Show_number_of_remaining_ads
: The Ad number countdown- Example: Ad 1 of 3, Ad 2 of 3, etc.
Customizing the Ad Overlay
You can customize the views and the default text associated with the Ad Overlay.
Modifying the View
To customize the Ad Overlay, you can replace your version of the ssai_ad_overlay.xml layout file in your app’s res folder. This file contains several different independent layouts, as shown here:
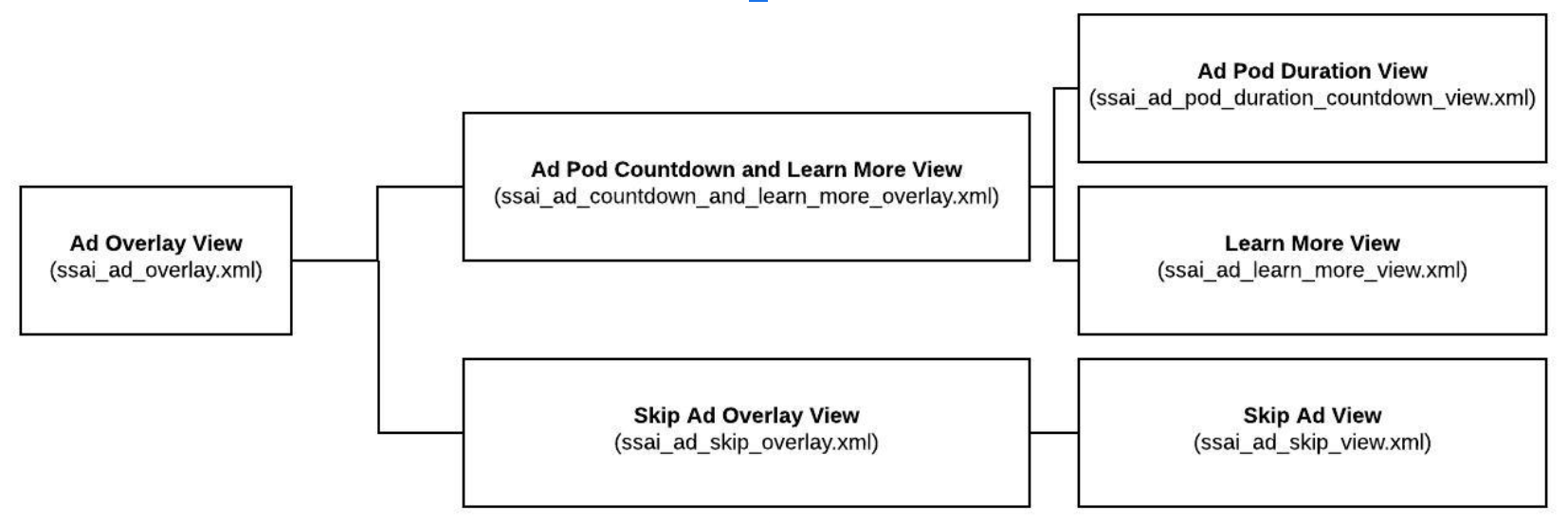
For example, you could override the Ad Pod duration view by updating the ssai_ad_pod_duration_countdown_view.xml file. You could also override the entire Ad Overlay View by replacing the ssai_ad_overlay.xml file.
This layout structure allows you to override particular components while maintaining backward compatibility with older SSAI plugin versions.
Explore the Ad Overlay files using Android Studio. As of version 6.9.0 of the Native SDK for Android, the SSAI plugin's ssai_ad_overlay.xml looks like this:
<?xml version="1.0" encoding="utf-8"?>
<FrameLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
tools:background="@color/white">
<include layout="@layout/ssai_ad_countdown_and_learn_more_overlay"/>
<include layout="@layout/ssai_ad_skip_overlay"/>
</FrameLayout>
When replacing a component xml file, you must use a TextView
with the original view Id specified to avoid unintended results. For details, see the following table
Component Name | Component Id | Component View |
---|---|---|
Ad Pod duration view | text_ad_countdown |
TextView |
Learn More view | text_ad_learn_more |
TextView |
Skip Ad view | text_ad_skip |
TextView |
Modifying the default text
All of the Ad Overlay components are TextViews
s defined in your app's string.xml file. To change the display text, override it by creating a string
or plurals
item using the same string id used by the Native SDK for Android.
The Ad Pod duration view uses the following strings:
<plurals name="ssai_message_ad_break_duration_countdown">
<item quantity="one">Your video will resume in\n%1$d second</item>
<item quantity="other">Your video will resume in\n%1$d seconds</item>
</plurals>
<string name="ad_info_now_text">Your video will resume now</string>
<string name="ad_buffering_text">Your ad is buffering …</string>
The Learn More view uses the following string:
<string name="ssai_message_learn_more">Learn More >> </string>
The Skip Ad view uses the following strings:
<plurals name="you_can_skip_text">
<item quantity="one">You can skip this ad in\n%d second</item>
<item quantity="other">You can skip this ad in\n%d seconds</item>
</plurals>
<string name="skip_text">Skip Ad</string>
Customizing the Ad Media Controller
You can customize the views and the default text associated with the Ad Media Controller.
Modifying the View
To customize the Ad Media Controller, you can replace your version of the default_ssai_ad_media_controller.xml layout file in your app’s res folder.
This overrides the default layout used in the SSAI plugin. Every time an Ad is detected, the SSAI plugin will switch to this layout and then return to the default controller when the Ad is finished.
When working with your own layout, use the same view type and view ids as originally specified to avoid unintended results. Explore the Ad Media Controller layout using Android Studio. For the SSAI plugin version 6.9.0, the default_ssai_ad_media_controller.xml looks like this:
<com.brightcove.player.mediacontroller.BrightcoveControlBar
xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:tools="http://schemas.android.com/tools"
android:id="@+id/brightcove_control_bar"
style="@style/SSAIAdControlBar"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_gravity="bottom"
android:background="@color/bmc_background"
android:orientation="vertical"
android:padding="8dp">
<LinearLayout
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:gravity="bottom"
android:orientation="horizontal">
<Button style="@style/BorderlessButton"
android:id="@id/play"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="@string/brightcove_controls_play"
android:visibility="gone"
tools:visibility="visible"/>
<include layout="@layout/ssai_ad_number_countdown_view"/>
<include layout="@layout/ssai_single_ad_duration_countdown_view"/>
<View
android:id="@id/two_line_spacer"
android:layout_width="0dp"
android:layout_height="0dp"
android:layout_weight="1"
android:visibility="gone"
tools:visibility="visible"/>
<Button style="@style/BorderlessButton"
android:id="@id/full_screen"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:padding="4dp"
android:text="@string/brightcove_controls_enter_full_screen"
android:visibility="gone"
tools:visibility="visible"/>
</LinearLayout>
</com.brightcove.player.mediacontroller.BrightcoveControlBar>
The SSAI Ad Media Controller component view types and view ids are described here:
Component Name | Component Id | Component View |
---|---|---|
Play button | play |
Button |
Full Screen button | full_screen |
Button |
Ad number countdown | text_ad_number_countdown |
TextView |
Single Ad duration countdown | text_single_ad_duration_countdown |
TextView |
Check out the Customized Controls Sample App, where the default media controller is customized to add new buttons. You can apply the same approach to customize your SSAI Ad Media Controller.
Modifying the default text
All of the Ad Media Controller components are TextViews
s defined in your app's string.xml file. To change the display text, override it by creating a string
or plurals
item using the same string id used by the Native SDK for Android.
The Ad number countdown view uses the following string:
<string name="ssai_message_ad_number_countdown">Ad %1$d of %2$d</string>
The Single Ad duration countdown view uses the following string:
<string name="ssai_message_ad_duration_countdown">(%1$s)</string>
Summary
You have a few options to customize the SSAI UI elements. The level of complexity will depend on what you want to achieve. From low to high level of complexity, you can:
- Configure/hide certain components through your VAST configuration
- Show/hide components using the
client_options
object in your ad configuration (currently Live SSAI only) - Change the string value displayed
- Override the UI layout