Overview
At a high level, a plugin integrates with the player by listening for and emitting events. A plugin can listen to events from the player and from other plugins. A plugin can emit events for the player and for other plugins.
This content along with sample code is located at https://github.com/BrightcoveOS/android-plugin-guide
Plugin architecture
A plugin should register with the SDK when they are instantiated. To register a plugin should emit a REGISTER_PLUGIN
event with a PLUGIN_NAME
property. For example:
Map<String, Object> properties = new HashMap<String, Object>();
properties.put(Event.PLUGIN_NAME, "my custom plugin");
eventEmitter.emit(EventType.REGISTER_PLUGIN, properties);
Video playback typically goes through a standard life cycle event flow:
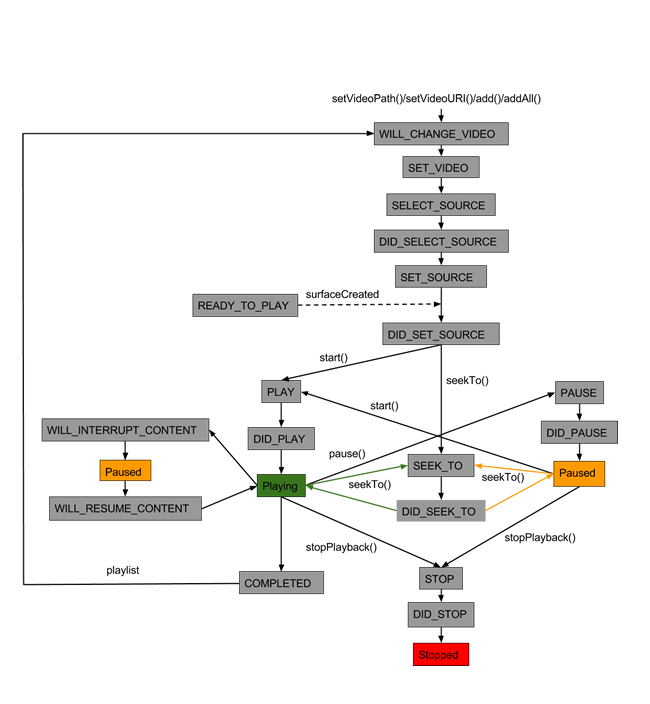
Event details
A plugin can listen for events, which initiate some action, in order to change the default behavior of the player. These events include:
WILL_CHANGE_VIDEO
SET_VIDEO
SET_SOURCE
PLAY
PAUSE
SEEK_TO
STOP
The default behavior can be changed by preventing the default listeners from receiving the event and/or by stopping propagation of the event to non-default listeners. Preventing the default listeners from receiving the event is accomplished by calling the preventDefault()
method. Event propagation can be stopped by calling the stopPropagation()
method. If only preventDefault()
is called, the rest of the non-default listeners will be notified. If only stopPropagation()
is called, the default listeners will still be notified, but the rest of the non-default listeners will be skipped. A plugin can also use these methods to pause the normal event flow and insert additional behavior, like initializing the plugin. A plugin can resume the event flow by emitting the original event again.
A plugin can also listen for events, which signal the completion of an action. These events are typically used by analytics plugins. The events include:
DID_CHANGE_LIST
DID_SELECT_SOURCE
DID_PAUSE
DID_PLAY
DID_SEEK_TO
DID_SET_SOURCE
DID_STOP
PROGRESS
COMPLETED
Interrupt playback
A plugin, which desires to interrupt the video content playback, should use WILL_INTERRUPT_CONTENT
and WILL_RESUME_CONTENT
. These events are typically used by advertising plugins. A plugin should emit WILL_INTERRUPT_CONTENT
to request that playback be suspended, if it is currently playing, and to request that the video view be made invisible. A plugin should emit WILL_RESUME_CONTENT
to request that the video view be made visible again. The WILL_RESUME_CONTENT
event should include an ORIGINAL_EVENT
property which will be emitted after the video view is made visible. The ORIGINAL_EVENT
should be a PLAY
event to resume playback, a CUE_POINT
event to continue cue point processing, or a COMPLETED
event to complete playback. A SKIP_CUE_POINTS
property should be added to the ORIGINAL_EVENT
to prevent recursive cue point processing.
Cue points
Many plugins will want to listen for CUE_POINT
events. There are three types of cue points, BEFORE
, POINT_IN_TIME
, and AFTER
. BEFORE
cue points are emitted just before playback begins. POINT_IN_TIME
cue points are emitted when playback reaches the cue point's position. AFTER
cue points are emitted just after playback completes. A plugin should use WILL_INTERRUPT_CONTENT
and WILL_RESUME_CONTENT
events to interrupt and resume content playback when handling a cue point event. In the case of before and after cue points, the event will include an ORIGINAL_EVENT
property, with either a PLAY
event or a COMPLETED
event. Cue point events also include a CUE_POINTS
property with the batch of cue points. START_TIME
and END_TIME
properties define the cue point time range.
Sample directory
The sample directory includes an Android Studio based project with two modules:
- SamplePlugin - An example plugin, which can be used as the basis for writing new plugins.
- SamplePluginApplication - Shows how plugins are incorporated into a Brightcove video application and can be used to test the SamplePlugin or new plugins.
Implementation steps
The steps to writing a plugin include:
- Copy the sample plugin to a new repository.
- Change the package and class names.
- Update the
TAG
initializer. - Edit the
@Emits
and@ListensFor
annotations to reflect the events which the plugin emits and listens for. - Modify the constructor as necessary.
- Replace or remove the
videoView
related logic as necessary. - Modify
initializeListeners()
by adding and/or subtracting event listeners as necessary. - Compile and distribute your plugin as an .aar file for Android Studio.