Overview
When you build Apple TV apps using the Brightcove Native SDK for tvOS, you will get a set of playback controls to allow users to navigate through video content, change the video's aspect ratio, get video information, and manage subtitles and audio settings.
The controls provided by the Brightcove Native SDK for tvOS include the following:
- Progress bar that can also show the clock time
- Top tab bar for video info, subtitles and audio
- Ability to add your own custom views and controls in the top tab bar
The controls and gestures used will be familiar to users, since they are similar to those found in the AVKit interface.
With Brightcove Server-Side Ad Insertion (SSAI), users will see a custom look during ad playback.
For details about using the Native SDK, see the Overview for the Brightcove Player SDK for tvOS document.
Supported versions
Apple TV UI controls are available with the following versions:
Device OS version
tvOS 9+
Native SDK version
Brightcove Native SDK for iOS version 6.3.0+
Siri Remote
The Siri Remote allows you to control your Apple TV. Here are some of the ways it can be used:
- Ask Siri to find what you want to watch.
- Use the Touch surface to move around the screen, swipe, highlight and select items.
- Press the control buttons to quickly play/pause content, adjust the volume and navigate to the home and menu screens.
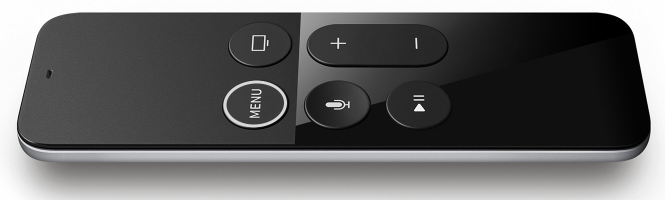
For more details, see Apple's Use your Siri Remote with Apple TV document.
Playback controls
Playback is controlled using gestures and button presses on the Apple Siri Remote. Below are some of the actions you can use with your app. For details, see the following:
Progress bar
A single tap on the Apple Siri Remote trackpad will show/hide the progress bar. When playback is paused, panning left and right will navigate backward and forward through your video.
For scrubbing, the user can click and hold, and then pan left or right to fast forward or reverse through a video.
While the video is playing, the user can double click on the left quarter of the Siri Remote trackpad to jump back 10 seconds or double click on the right quarter of the trackpad to jump forward 10 seconds.

Video aspect ratio
A double tap on the Apple Siri Remote trackpad will cycle through three standard video aspect ratios:
- Letterboxing to respect aspect ratio (full video displayed)
- Full screen with aspect ratio respected (video may be cropped)
- View stretched to fit full screen (aspect ratio ignored)
Top tab bar
Swiping down on the Apple Siri Remote trackpad will display the top menu and hide the progress view. Once visible, swiping up will hide the top menu.
The tab bar has the following default items:
- Info
- Subtitles
- Audio
You can modify the text labels associated with each tab bar item, or you can create your own custom views in the tab bar.
Info
Selecting the Info top menu item displays the title, description and length associated with the currently loaded video. This data is retrieved from the video's metadata in the Playback API, but you can set your own text when the video loads.
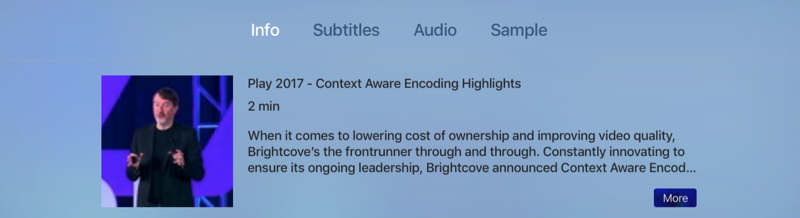
Subtitles
Selecting the Subtitles top menu item displays options for showing captions associated with the video. Here, you can manage the subtitles/captions to show during playback.

Audio
Selecting the Audio top menu item displays the audio options available during video playback.

Custom
With the Brightcove Native SDK for tvOS, you can easily add your own views in the tab bar. Your views can contain any standard UIKit views or controls that you need.
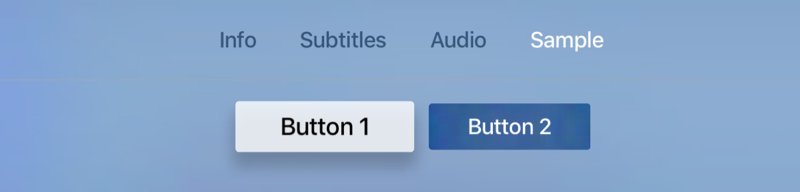
Advertising with SSAI
In addition to content playback, we've added an advertising mode when using Brightcove Server-Side Ad Insertion (SSAI). This mode includes the following features:
- Ability to prevent the user from scrubbing past ads
- Display feedback on the time and ads remaining in an ad break

Sample
To experience the Apple TV controls and see how to create a custom tab bar item view, check out the following code sample:
Live/Live DVR
When working with your Apple TV app, the Brightcove Native SDK for tvOS supports both live and DVR live streams. DVR live enables users to do the following:
- Use the progress bar to seek to past moments
- Jump back to the current live moment to watch content in real-time
Implementing playback
To play a live or live DVR stream from your Apple TV app, do the following:
- Start with the basic Apple TV sample code. Open the project in Xcode and open the ViewController.swift file.
-
In the
createTVPlayerView()
function, set theplayerType
to eitherlive
orliveDVR
.playerView = BCOVTVPlayerView(options: options) if (playerView != nil) { playerView!.frame = self.videoContainerView.bounds playerView!.playerType = BCOVTVPlayerType.liveDVR; self.videoContainerView.addSubview(playerView!) }
- Locate the
viewDidLoad()
function. - The call to the
createSampleTabBarItemView()
function is optional and shows you how to create a custom view in the tab bar. For this example, we will comment the call to this function. - Comment the call to the
requestContentFromPlaybackService()
function. -
In the
viewDidLoad()
function, before the closing bracket, add code to do the following:override func viewDidLoad() { super.viewDidLoad() // Do any additional setup after loading the view, typically from a nib. createTVPlayerView() // createSampleTabBarItemView() // Create and configure the playback controller playbackController.delegate = self playbackController.isAutoAdvance = true playbackController.isAutoPlay = true // Link the playback controller to the Player View playerView?.playbackController = playbackController // requestContentFromPlaybackService() // live stream let url = URL(string: "your live stream url") let video: BCOVVideo = BCOVVideo(hlsSourceURL:url ) self.playbackController.setVideos([video] as NSArray) }
- Define the URL string for your live or live DVR stream.
- Create a
BCOVVideo
object using your live or live DVR stream URL from the previous step. - Call the
setVideos()
function to add your live stream to theplaybackController
's playback queue, which is set to automatically start playing.
Live stream indicator
The progress bar provided by the Native SDK for tvOS will not be filled in for live streams. An indicator below the progress bar will inform the user when the stream is live and when it is paused:

Live DVR progress bar
An indicator below the progress bar will inform the user when the stream is live and when it is paused.
While watching the live stream in real-time, the progress bar will be completely filled. Seeking to a previous time in the stream will look similar to this:
