Overview
Server-Side Ad Insertion (SSAI) allows you to embed ads into your videos so that they can't be blocked by ad blockers in the browser. Dynamic Delivery is the next generation ingest and delivery system which reduces your storage footprint and dynamically packages media. Learn more about using Dynamic Delivery for your videos.
SSAI works with both DRM and non-DRM content.
Any video that you retrieve from Video Cloud that has been ingested with Dynamic Delivery, will include the ads specified in the VMAP file in your ad configuration.
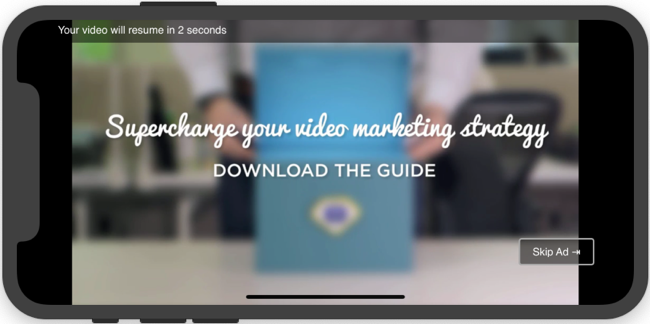
To play server-side ads with your video content stored in Video Cloud, follow these steps:
Create an ad configuration
The ad configuration defines various aspects of SSAI playback, including a URL to your Video Multiple Ad Playlist (VMAP) file, beacons, and other configurations. To create an ad configuration, follow these steps:
-
In Video Cloud Studio, expand the ADMIN menu and select Server-Side Ad Settings.
Ad configuration admin -
Add information for your ad configuration and select Save.
Ad settings
For details, see the Configuring Server-Side Ad Settings document.
To create an ad configuration using the SSAI API, see the Video Cloud SSAI Ad Config API document.
Android implementation
Follow these steps within your app to get your ad configuration and play your video:
- In Android Studio, open your MainActivity.java file.
- Add the following import statements:
import com.brightcove.ssai.SSAIComponent; import com.brightcove.player.network.HttpRequestConfig;
- Define constants for your account id, policy key, video id, and your ad configuration id.
private String accountId = "your account id"; private String policyKey = "your policy key"; private String videoId = "your video id"; private String adConfigId = "your ad configuration id";
- Create an instance of the catalog service, which provides asynchronous methods for retrieving data from the Playback API. Create an instance of the SSAI component.
Catalog catalog = new Catalog(eventEmitter, myAccountId, myPolicyKey); SSAIComponent plugin = new SSAIComponent(appContext, getBrightcoveVideoView());
- Set the adConfigId as a URL parameter.
HttpRequestConfig httpRequestConfig = new HttpRequestConfig.Builder() .addQueryParameter("ad_config_id", myAdConfig) .build();
- Call the catalog service to retrieve your video along with your ad configuration from the Playback API. Start video playback with your specified ads.
catalog.findVideoByID(myVideoId, httpRequestConfig, new VideoListener() { @Override public void onVideo(Video video) { // Process the Video plugin.processVideo(video); } });
Captions
Events
For details about events associated with Server-side ad insertion, see the following:
Seek without ads
When you want to disable ad playback while the user is seeking through the video, refer to the Seek without ads code snippet.
Code sample
Here is a complete code sample:
iOS implementation
Follow these steps within your app to get your ad configuration and play your video:
- In Xcode, open your ViewController.m file.
- Import the SSAI plugin for iOS.
import BrightcoveSSAI
- Define constants for your account id, policy key, video id, and your ad configuration id.
struct Constants { static let AccountID = "your account id" static let PlaybackServicePolicyKey = "your policy key" static let VideoId = "your video id" static let AdConfigId = "your ad configuration id" }
- Define the
BCOVPlaybackService
class, which provides asynchronous methods for retrieving data from the Playback API.private lazy var playbackService: BCOVPlaybackService = { let factory = BCOVPlaybackServiceRequestFactory(accountId: Constants.AccountID, policyKey: Constants.PlaybackServicePolicyKey, baseURLStr: "https://edge.api.brightcove.com/playback/v1") return BCOVPlaybackService(requestFactory: factory) }()
- Set the query parameter value to your ad configuration id.
let queryParameters = [kBCOVPlaybackServiceParamaterKeyAdConfigId: Constants.AdConfigId]
-
Call the
playbackService
to retrieve your video along with your ad configuration from the Playback API. Start video playback with your specified ads.playbackService.findVideo(withVideoID: Constants.VideoId, parameters: queryParameters) { [weak self] (video: BCOVVideo?, jsonResponse: [AnyHashable: Any]?, error: Error?) -> Void in guard let _video = video else { print("ViewController Debug - Error retrieving video: \(error?.localizedDescription ?? "unknown error")") return } self?.playbackController?.setVideos([_video] as NSFastEnumeration) }
Events
For details about events associated with Server-side ad insertion, see the following:
Seek without ads
When you want to disable ad playback while the user is seeking through the video, refer to the Seek without ads section of the SDK reference.
Code sample
Here is a complete code sample:
Related topics
For more details, see the following:
tvOS implementation
The code to implement SSAI with the Native SDK for tvOS is similar to the iOS implementation above.
Events
For details about events associated with Server-side ad insertion, see the following:
Code sample
Here is a complete code sample: