Overview
Picture-in-Picture mode (PIP) allows users to multitask on their devices. With this feature, you can create apps where a small video playback window is pinned to a corner of the screen while the user performs other tasks.
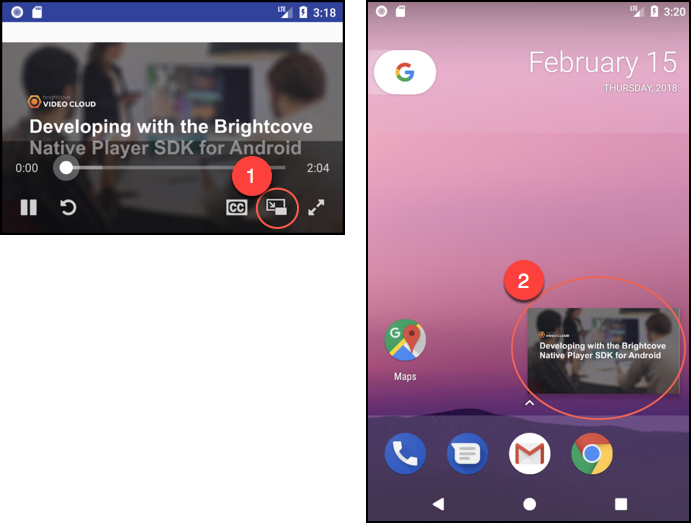
Features
The Picture-in-Picture functionality provided by the Brightcove Native SDKs, supports the following features:
- Where a user enters Picture-in-Picture mode by clicking on the PIP icon in the control bar, a developer can enter PIP mode programmatically.
- The user can drag the PIP window around the corners of the screen to place it in the most convenient location.
- The user can pause and play the video in the PIP window, or remove it completely. The user can also maximize the video back to the in-app experience.
- PIP mode works on top of the app, the device background and other applications.
Supported versions
Picture-in-Picture mode is supported with the following versions:
Device OS version
- Android: Oreo 8.0+ (API level 26+)
- iOS: 10.0+
Native SDK version
- Android: Brightcove Native SDK for Android version 6.1.0+
- iOS: Brightcove Native SDK for iOS version 6.4.4+
Android implementation
The following topics will help you get started with the Picture-in-Picture feature:
For a code example, see the PictureInPictureSampleApp.
For more details, see Android's Picture-in-Picture support guide.
Using BrightcovePlayer
The easiest way to start using Picture-in-Picture mode is to have your activity extend the BrightcovePlayer
. To do this, follow these steps:
- In your styles.xml file, enable the Picture-in-Picture button by adding the following code:
<style name="BrightcoveControlBar" parent="BrightcoveControlBarDefault"> <item name="brightcove_picture_in_picture">true</item> </style>
- In your AndroidManifest.xml file, declare Picture-in-Picture support for the activity by adding the following:
<activity android:name="VideoActivity" android:resizeableActivity="true" android:supportsPictureInPicture="true" android:configChanges= "screenSize|smallestScreenSize|screenLayout|orientation" ...
This is an important step. If you do not declare it, when a user presses the Picture-in-Picture button in the Brightcove Media Controller, an
IllegalStateException
will be thrown:java.lang.IllegalStateException: enterPictureInPictureMode: Current activity does not support picture in picture
When going into Picture-in-Picture mode, the activity itself is resized, retaining all of the activity components in the Picture-in-Picture window. To avoid this and to provide an easier implementation, the Brightcove video view will go into Full Screen automatically to match the parent layout width and height showing only the video in the Picture-in-Picture window. Once you leave this mode, Brightcove video view will return to its original state.
If you do not want Picture-in-Picture mode in your app, you can disable the Picture-in-Picture button by adding the following code in your styles.xml file:
<style name="BrightcoveControlBar" parent="BrightcoveControlBarDefault">
<item name="brightcove_picture_in_picture">false</item>
</style>
Using a regular Activity
If you are using an activity which does not extend BrightcovePlayer
, in addition to declaring support for Picture-in-Picture mode as mentioned in the previous section, you need to follow a few extra steps:
- Open the MainActivity.java file.
- In the
onCreate
method of the activity, register the Activity with the BrightcovePictureInPictureManager
:PictureInPictureManager.getInstance().registerActivity(Activity, BrightcoveVideoView)
- In the
onDestroy
method, unregister the Activity:PictureInPictureManager.getInstance().unregisterActivity(Activity)
- Override the
onPictureInPictureModeChanged
Activity method, and callonPictureInPictureModeChanged()
method as shown below:@Override public void onPictureInPictureModeChanged (boolean isInPictureInPictureMode, Configuration newConfig) { super.onPictureInPictureModeChanged(isInPictureInPictureMode, newConfig); PictureInPictureManager.getInstance().onPictureInPictureModeChanged(isInPictureInPictureMode, newConfig); }
- Finally, override the
onUserLeaveHint()
Activity method, and callonUserLeaveHint()
as shown below:@Override public void onUserLeaveHint () { super.onUserLeaveHint(); PictureInPictureManager.getInstance().onUserLeaveHint(); }
After following these steps, the Picture-in-Picture button will appear in the Brightcove Media Controller automatically. You can also trigger Picture-in-Picture mode programmatically as follows:
PictureInPictureManager.getInstance().enterPictureInPictureMode()
Customizing Picture-in-Picture
You can customize the behavior and appearance of the Picture-in-Picture window through the PictureInPictureManager
. For example, you can change the aspect ratio of the window or enable/disable captions for your videos.
Android Oreo natively provides three parameters that can be customized:
- aspect ratio
setAspectRatio(Rational)
- source bounds hint
setSourceRectHint(Rect)
- list of remote actions
setUserActions(List<RemoteAction>)
User actions
Brightcove adds a RemoteAction
by default, which handles the Play/Pause
video events and sets their respective icons. This means that the default RemoteAction
will be added to the list of remote actions you set with the following:
setUserActions
Keep in mind that you can only set a limited number of actions. To get the maximum number of actions, call the following:
Activity.getMaxNumPictureInPictureActions()
Closed captions
The Native SDK for Android provides an API to enable captions in PiP mode and set a reduction scale factor (sets the PiP caption size to be a percentage of the caption size in a non-PiP player).
You can enable/disable Closed Captions when playing a video in Picture-in-Picture mode by calling the following in the inPictureInPictureManager
.
setClosedCaptionsEnabled(boolean)
Closed Captions are disabled by default in phones but enabled in tablets. To reduce the size of the Closed Captions while playing in Picture-in-Picture mode, call the following to set a value between 0
and 1.0f
.
setClosedCaptionsReductionScaleFactor(float)
By default, the Closed Captions size in Picture-in-Picture mode is 0.5f
or 50% the original size. Lastly, to enter Picture-in-Picture mode when the Activity onUserLeaveHint()
is called, set the following to true
:
setOnUserLeaveEnable(boolean)
Parameters
In order to retrieve the current Picture-in-Picture parameters, you can call the following:
PictureInPicture.getBrightcovePictureInPictureParams()
This call returns the BrightcovePictureInPictureParams
object, which has the getters for all the parameters previously discussed.
iOS implementation
To get started with Picture-in-Picture mode, add showPictureInPictureButton
to BCOVPUIPlayerViewOptions
. When set to true, a Picture-in-Picture button will be added to the player controls on supported devices.
Picture-in-Picture mode is one of the new features with iOS 14.
For more details, see the following:
- The Picture in Picture section of the Brightcove Native SDK for iOS reference
- Apple's Adopting Picture in Picture in a Custom Player document
For a code example, see the Basic Video Playback App.
Limitations
Android
- Picture-in-Picture mode will only work on devices with Android Oreo or higher
- It is currently not supported for Android TV
- Videos with ads are not supported
- SSAI nor CSAI ads are supported
- 360 videos are not supported
iOS
- Picture-in-Picture mode is supported with Brightcove's server-side ads (SSAI), but PIP is not supported with client-side ads, including IMA, FreeWheel, or Pulse.
- 360 videos are not supported
Known issues
Android
- We found that Widevine-protected videos playing in Picture-in-Picture mode will turn transparent or black in the Pixel C with Android 8.0. The issue is not reproducible in the same device with Android 8.1.