Overview
The Brightcove Native SDK for Android is available in a new major version bringing important architecture changes and playback enhancements, including:
- Support for audio-only content
- Media-Style notification with customizable playback controls from the notification shade and lockscreen
- Support for an on-going foreground service for continuous playback
- Improved loading playlists and pre-buffering media for a smoother end-user experience
Audio-only content
The Brightcove Native SDK for Android supports audio-only playback and rich playback controls. Audio playback will continue to play even if the host application is in the background, giving the end-user more control of their listening experience. Support for audio playback has been integrated with the current Native SDK for Android APIs to ensure backward compatibility with all of Brightcove's features.
For implementation details, see the Audio Only with the Native SDKs document.
SDK changes
With this release of the Native SDK for Android, the following classes were added to support continuous integration with background playback, ExoPlayer, and media style notifications:
MediaPlayback<Player>
BrightcoveNotification
MediaPlaybackService
MediaPlayback<Player>
With the changes in the Native SDK for Android version 8.0.0, Brightcove has decoupled playback business logic out of the View
and Activity
. This business logic now lives in the new MediaPlayback
class, enabling media to persist beyond the Activity
lifecycle using a ForegroundService
. The playback status is shared across the notification and its corresponding View (when the app is in the foreground).
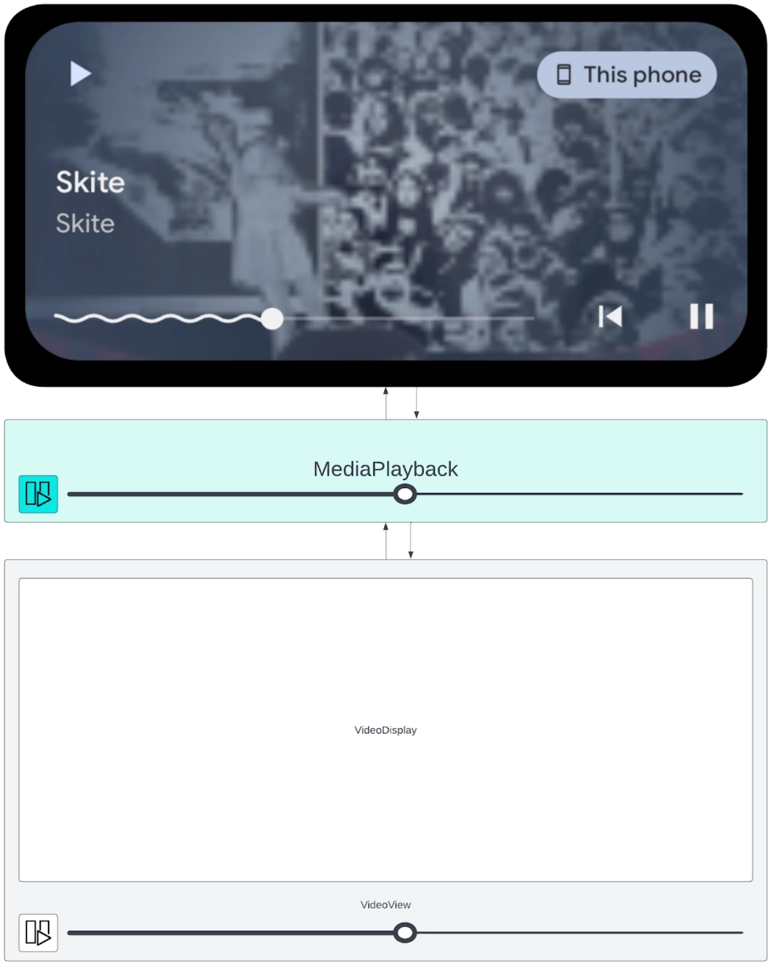
Brightcove has made significant improvements in the utilization of ExoPlayer's internal playlist support. Previously, a new instance of ExoPlayer was created for each media item loaded into the SDK.
Now, media playback enhances pre-buffering the MediaSource
for ExoPlayer. This improves performance and employs ExoPlayer's Player.Listener
to emit important playback states.
Support for foreground and background playback will merge a FOREGROUND_SERVICE
permission to your AndroidManifest file during your app build process. This permission is a requirement with SDK v8.0.0.
BrightcoveNotification
Users can interact with the player from the notification and lock screen using action buttons. By default, the notification includes play, pause, next, and previous action buttons.
You can customize the action buttons by retrieving the notification instance and setting a config
.
BrightcoveExoPlayerVideoView player = findViewById(R.id.player_view);
PlaybackNotification.Config config = new PlaybackNotification.Config(this)
.setUsePlayPauseActions(true)
.setUseNextAction(false)
.setUsePreviousAction(false)
.setUseFastForwardAction(true)
.setUseRewindAction(true);
player.getPlayback().getNotification().setConfig(config);
The following table shows the default values and descriptions of the action buttons:
Method Name | Default Value | Description |
---|---|---|
usePlayPauseActions |
True |
Sets whether the play and pause actions are used |
useRewindAction |
True |
Sets whether the rewind action is used |
useRewindActionInCompatView |
False |
If useRewindAction is true, sets whether the rewind action is also used in compact view (including the lock screen notification); Else does nothing |
useFastForwardAction |
True |
Sets whether the fast forward action is used |
useFastForwardActionInCompactView |
False |
If useFastForwardAction is true, sets whether the fast forward action is also used in compact view (including the lock screen notification); Else does nothing |
usePreviousAction |
True |
Whether the previous action is used |
usePrevioiusActionInCompatMode |
False |
If usePreviousAction is true, sets whether the previous action is also used in compact view (including the lock screen notification); Else does nothing |
useNextAction |
True |
Whether the next action is used |
useNextActionInCompactView |
False |
If useNextAction is true, sets whether the next action is also used in compact view (including the lock screen notification); Else does nothing |
useStopAction |
False |
Sets whether the stop action is used |
Notification metadata
By default, the notification uses the asset's metadata and creates an implied PendingIntent
to recreate your activity. If you need further control over metadata displayed in the notification, you may implement a MediaDescriptionAdapter
:
new PlaybackNotification.Config(this)
.setAdapter(new PlaybackNotification.MediaDescriptionAdapter() {
@Override
public CharSequence getCurrentContentTitle(
MediaPlayback playback
) {
// TODO: return the current content title
}
@Override
public Bitmap getCurrentLargeIcon(
MediaPlayback playback,
BitmapCallback callback
) {
// TODO: return the bitmap for currently playing item
}
@Nullable
@Override
public PendingIntent createCurrentContentIntent(
MediaPlayback<?> playback
) {
// TODO: return your custom PendingIntent
}
@Nullable
@Override
public CharSequence getCurrentContentText(
MediaPlayback<?> playback
) {
// TODO: return the current content text
}
@Nullable
@Override
public CharSequence getCurrentSubText(
MediaPlayback<?> playback
) {
// TODO: return the current sub-text.
}
}
);
MediaPlaybackService
The Native SDK for Android supports notifications designed for media playback. The default player behavior when playing an audio asset is to start a new ForegroundService with an ongoing playback notification with media controls. The media controls are displayed below the Quick Settings panel and on the lock screen:
The media controls are found on the lock screen and below the Quick Settings panel:
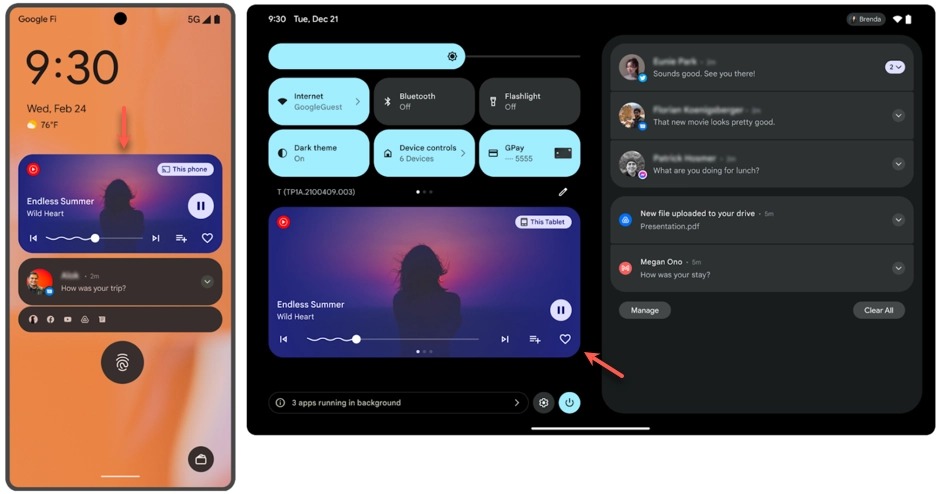
The notification lets users view and control playback when the host application is in the background. The Native SDK automatically handles:
- Loading album artwork and metadata
- Action buttons (play/pause, next, previous, fast-forward, rewind, and stop)
- Activity lifecycle events
You can customize the notification style and media controls using the PlaybackNotification.Config
class.