Introduction
The Native SDK for Android uses an event-driven model, which listens for user actions with the video player.
Event-driven model
In traditional programming models, the flow is static and pre-defined by the programmer. In an event-driven model, there is no pre-set flow. Instead, the flow is determined by events, such as user actions. There is usually a main loop that listens for events and triggers callback functions.
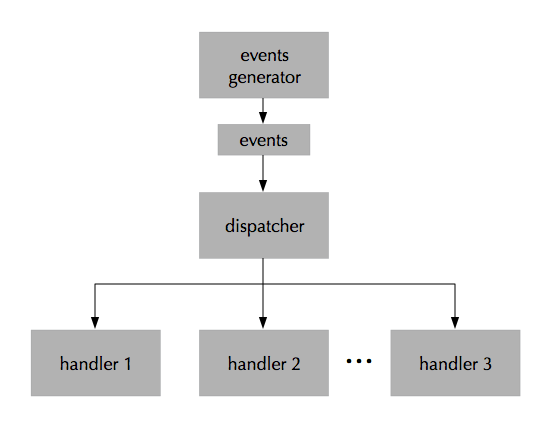
Events
While using the Brightcove Player SDK for Android, you can take advantage of pre-defined events to control functionality in your app. Because events are just string literals, you can easily create and use your own events.
Events in the Brightcove Player SDK for Android represent a state or action that can be passed down through a chain of listeners. All events have a type and a map of properties associated with them. Events can be stopped from propagating down the chain of listeners, or can be instructed to prevent their default listeners in the video playback classes.
Events can represent state or an action occurring. For example, the event type DID_SEEK_TO
represents the state of the action SEEK_TO
.
For a complete list of pre-defined events, select the following image:
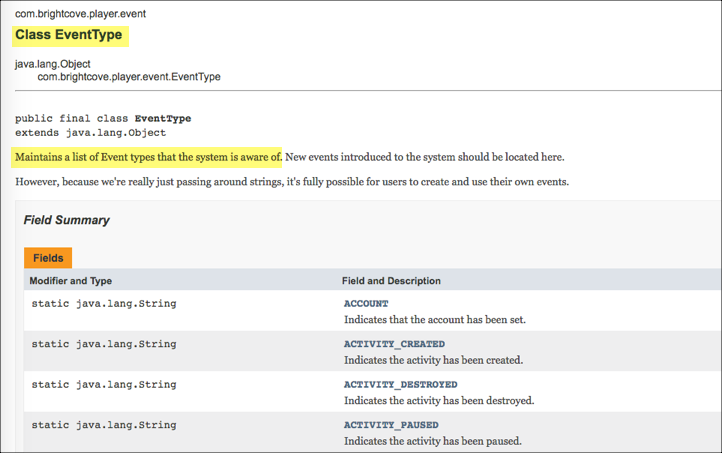
EventEmitter
The Brightcove BaseVideoView is designed as a drop-in replacement for Android's native VideoView. This class also starts the EventEmitter which ties all of the SDK components together. When a user interacts with the video view, the EventEmitter
emits an event, such as play, pause, etc. Components interested in each event can listen for and act upon them.
Plugins can be added which listen and emit events when they want the SDK to do certain things.
The Native SDK provides pre-defined events to control your app, but you can easily create and use your own events.
It is also possible to create your own event emitter and instruct the BrightcoveExoPlayerVideoView to use it. This is useful if you want to control a chain of listeners.
Components and events
For each component in the Brightcove SDK for Android, you can find all of the events that are associated with it. To do this, follow these steps:
- Navigate to the Brightcove Player SDK for Android reference.
- In the left-side menu, select a component.
- In the component class details section, you will find a list of events that are emitted and listened for by this component.
Components and events
Common playback events
These diagrams show some of the common playback events and how they interact with the components in the Brightcove Player SDK for Android.
add()/set() method events
This diagram shows events related to the add(Video)
, addAll(Collection)
, setVideoPath()
, and setVideoURI()
methods.
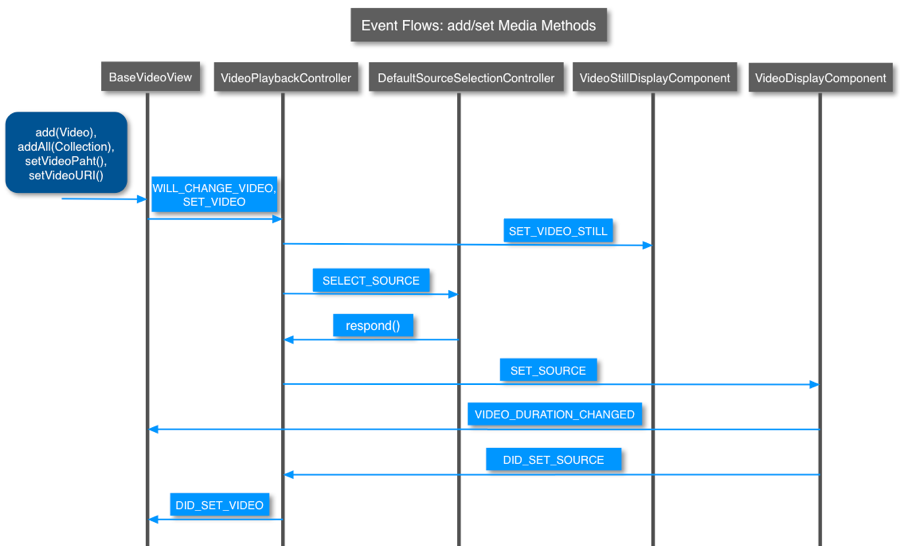
start() method events
This diagram shows events related to the start()
method.
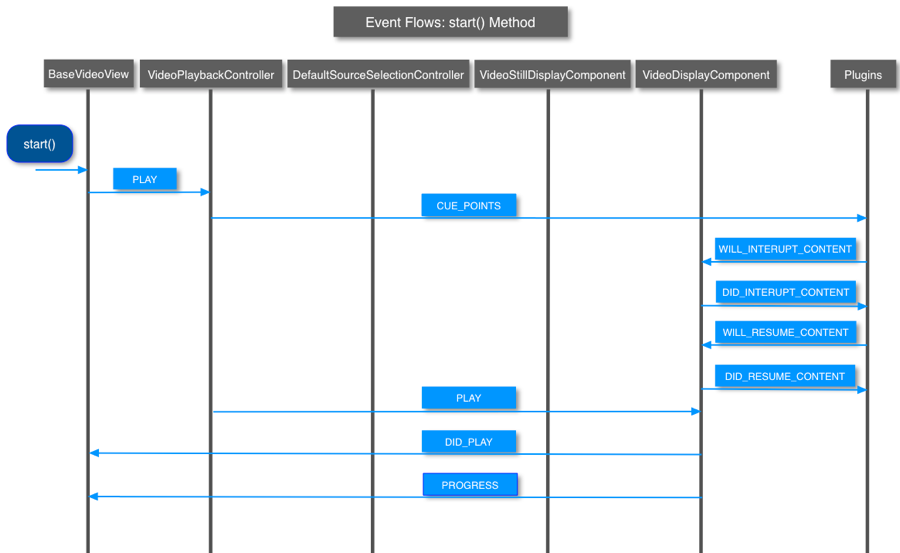
seekTo() method events
This diagram shows events related to the seekTo()
method.
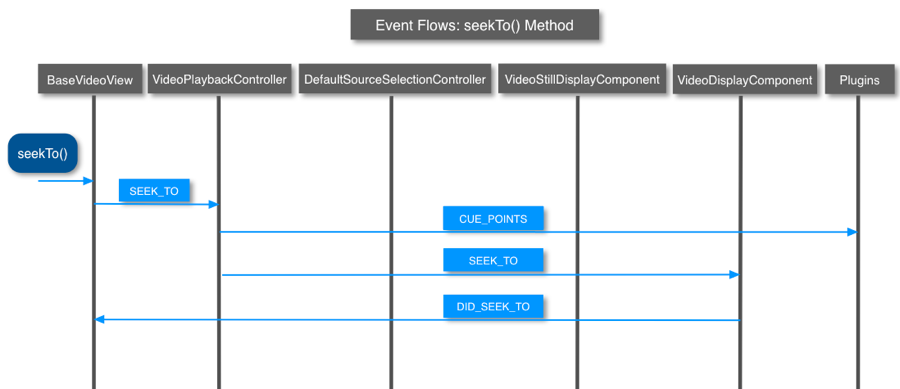
pause() method events
This diagram shows events related to the pause()
method.
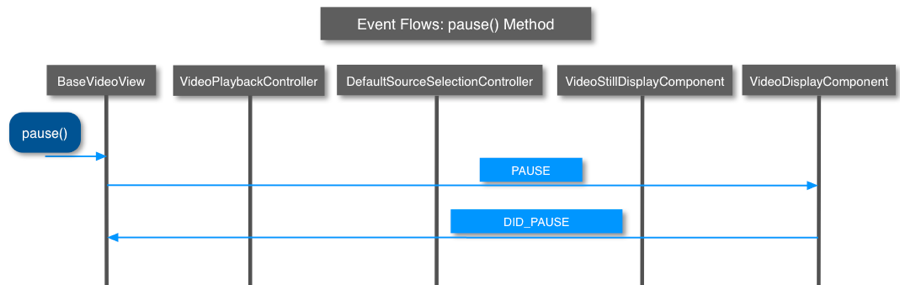
stopPlayback() method events
This diagram shows events related to the stopPlayback()
method.
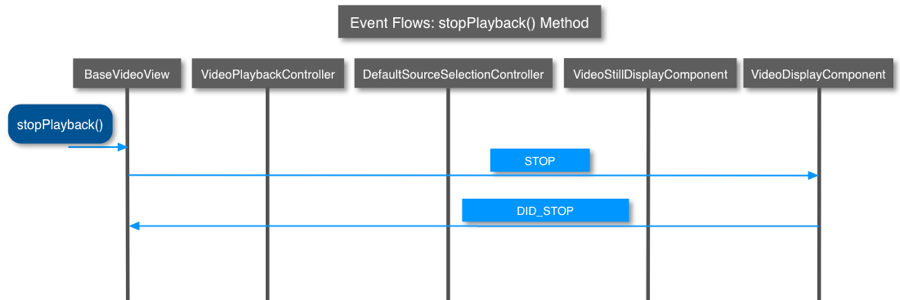
Common ad events
In your app, you can listen and act upon a set of advertising events from the Brightcove Player SDK for Android.
IMA ad events
This diagram shows the ad events for the Google IMA advertising plugin. These ad events are listened for within the exoplayer sample: AdRulesIMASampleApp.
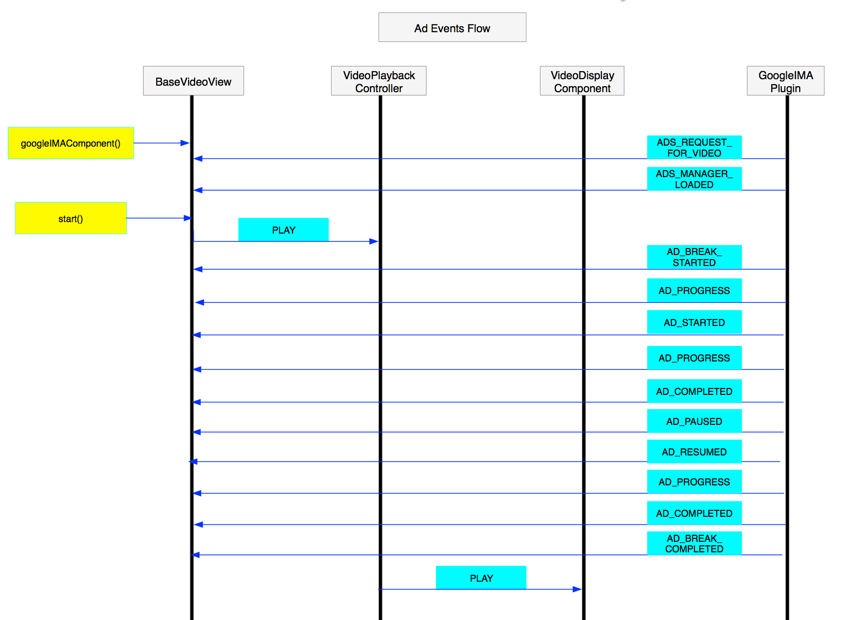
Common ad events
Here is a list of the common advertising events from the Brightcove Player SDK for Android that you can listen for and act upon:
Code Example
When an Ad break starts, the EventType.AD_BREAK_STARTED
event is emitted. When an Ad break ends, the EventType.AD_BREAK_COMPLETED
is emitted.
You can listen and react to events as shown here:
eventEmitter.on(EventType.AD_BREAK_STARTED, new EventListener() {
@Override
public void processEvent(Event event) {
// React to the event
}
});
eventEmitter.on(EventType.AD_BREAK_COMPLETED, new EventListener() {
@Override
public void processEvent(Event event) {
// React to the event
}
});
You can emit events by doing the following:
eventEmitter.emit(EventType.STOP);
You now have a basic understanding of the Brightcove Player SDK for Android. Next, you can walk through the steps of building an app which uses the Player SDK.