Overview
Android Pie is the newest Android OS and was officially released on August 6th 2018. For feature details, see the Android Pie Features document. Android Pie includes several behavior changes. Most of them affect apps which target Android Pie (API level 28), but you may also find issues with apps targeting an older Android version, running on an Android Pie device.
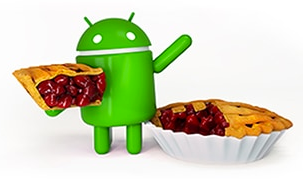
There are a couple of changes to be aware of when using the Brightcove SDK for Android:
For a full list of behavioral changes for your apps, see the Behavior Changes for all apps document. For behavioral changes exclusive when targeting Android Pie, see the Behavior Changes for API 28+ document.
TLS enabled by default
Transport Layer Security (TLS) is a protocol for providing secure communications over a computer network. TLS is implemented on top of HTTP, allowing for encrypted communication over HTTPS.
When Google released Android Marshmallow (API level 23), it provided a configuration to disable clear text traffic, which would prevent your app from making clear HTTP requests. With the release of Android Pie (API level 28), clear text traffic is disabled by default.
With clear text traffic disabled by default, if your application attempts to do a clear HTTP request, an IOException is thrown with the following message:
Exception: IOException java.io.IOException: Cleartext HTTP traffic to * not permitted
For more information, visit google’s website: Framework security changes and Network security configuration.
If your app targets Android Pie (API level 28) or above, you need to handle this behavior change so that your app works as expected. You have three options:
- Ensure your app only makes HTTPS requests
- Enable clear HTTP traffic for all domains
- Enable clear HTTP traffic for specific domains
HTTPS only
One option is to make sure all of your network requests use HTTPS. To do so, follow these steps:
-
Enable your CDN for secure communication.
- Video Cloud customers (whether you are using the house CDN or a named CDN) should contact their Brightcove account managers to ensure accounts are configured to deliver over HTTPS.
-
For those using remote assets, you may need to configure your CDN appropriately.
-
Use secure communication to deliver your media content. This includes video, ads, captions, poster images, thumbnails and other third-party API connections.
-
Video Cloud customers: Use the Playback API to retrieve the secure protocol for each of your assets. The Brightcove SDK for Android selects HTTPS by default when available.
- Brightcove Player customers: Make sure your media content is delivered over HTTPS.
-
HTTP for all domains
Your app can use clear HTTP traffic for all domains. Here are two examples for how you can do this:
Update the application tag
-
To enable this, open the AndroidManifest file, and add the following line in the
application
tag. For example:<application android:name="mypackage.MyApplication" android:usesCleartextTraffic="true"> … </application>
Add a network security configuration file
-
Create an xml file, for example network_security_config.xml, and add it to the res/xml directory. See the following example for how to allow clear traffic:
<network-security-config> <base-config cleartextTrafficPermitted="true"/> </network-security-config>
-
Set the file name in your AndroidManifest as shown below:
<application android:name="mypackage.MyApplication" android:networkSecurityConfig="@xml/network_security_config"> … </application>
For more details, see Android's Network security configuration document.
HTTP for specific domains
You can specify certain domains to be permitted to use clear HTTP requests. To do this, follow these steps:
-
Create an xml file, for example network_security_config.xml, and add it to the res/xml directory. See the following example for how to set your domains:
<network-security-config> <domain-config cleartextTrafficPermitted="true"> <domain includeSubdomains="true">c.brightcove.com</domain> <domain includeSubdomains="true">solutions.brightcove.com</domain> </domain-config> </network-security-config>
-
Set the file name in your AndroidManifest as shown below:
<application android:name="mypackage.MyApplication" android:networkSecurityConfig="@xml/network_security_config"> … </application>
For more details, see Android's Network security configuration document.
Apache HTTP client deprecation
With Android Pie, Apache HTTP client support has been removed. This affects apps that target Android Pie or higher. It might also affect apps that target older versions of Android when using a non-standard ClassLoader
. At runtime, if the ClassLoader
attempts to load an Apache HTTP client class on Android Pie, it will throw a NoClassDefFoundError
. For more information, see Google’s : Apache HTTP client deprecation document.
If your app is using the Brightcove FreeWheel plugin, you will experience this problem and get an exception as shown here:
java.lang.NoClassDefFoundError: tv.freewheel.utils.URLLoader
Caused by: java.lang.NoClassDefFoundError: Failed resolution of: Lorg/apache/http/impl/client/BasicCookieStore;
In order to fix this problem, include the following line to your app’s manifest file:
<uses-library android:name="org.apache.http.legacy" android:required="false"/>
Deprecated constants
There are several constants that were deprecated in the ConnectivityManager class. Some of these constants are currently used by the Brightcove SDK, specifically by the Offline Playback and the Offline Analytics features. Here is the list:
android.net.ConnectivityManager.TYPE_BLUETOOTH
android.net.ConnectivityManager.TYPE_ETHERNET
android.net.ConnectivityManager.TYPE_MOBILE
android.net.ConnectivityManager.TYPE_VPN
android.net.ConnectivityManager.TYPE_WIFI
android.net.ConnectivityManager.TYPE_WIMAX
This doesn't affect any functionality on the mentioned features, but be aware that these constants might be removed in a future Android OS version. This also applies to the Brightcove Native SDK.