Introduction
Brightcove's Player Enhancements for Live SSAI allows you to improve the ad breaks in live streams with ad counts, ad countdown timers, click through ads and companion ads. These live streams can be played using the Brightcove Native SDKs.
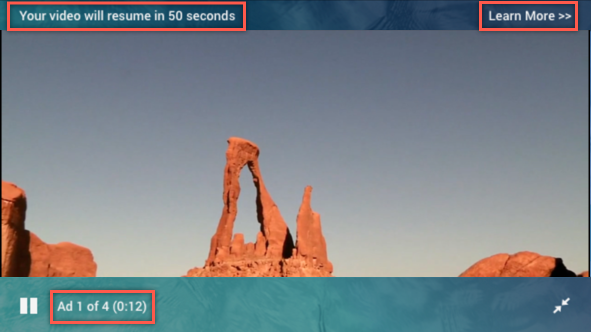
Since TVs do not support a web browser, companion and click-through ads are not available on connected TVs.
If you are new to this feature, see the following:
Steps
To play live streams with an enhanced player using the Brightcove Native SDKs, follow these steps:
- Follow the workflow in the Implementing Player Enhancements for Live SSAI document, up through creating your playback token.
-
Build your app:
- Continue with the workflow in the the Implementing Player Enhancements for Live SSAI document.
Android implementation
You will need the following values to build your app using the Native SDK for Android:
- Playback token
- Account Id
- Video Id
- Policy Key
To get your Policy Key, review the Policy Keys document.
Follow these steps to build your app:
- Use the Basic SSAI Sample App as a starting point for your code.
- In the res/values/strings.xml file, replace the following with your own values:
- Account Id
- Video Id
- Policy Key
-
In the MainActivity.java file, replace the
ad_config_id
value with your playback token.private final String AD_CONFIG_ID_QUERY_PARAM_VALUE = "your playback token";
-
The
ad_config_id
with your playback token value is added to the HTTP request as a query parameter.HttpRequestConfig httpRequestConfig = new HttpRequestConfig.Builder() .addQueryParameter(AD_CONFIG_ID_QUERY_PARAM_KEY, AD_CONFIG_ID_QUERY_PARAM_VALUE) .build();
-
Make the catalog call to the Playback API with your live stream video id and the updated HTTP request.
catalog.findVideoByID(getString(R.string.video_id), httpRequestConfig, new VideoListener() { @Override public void onVideo(Video video) { plugin.processVideo(video); } });
- Your player is ready to play your live stream.
iOS implementation
You will need the following values to build your app using the Native SDK for iOS:
- Playback token
- Account Id
- Video Id
- Policy Key
To get your Policy Key, review the Policy Keys document.
Follow these steps to build your app:
- Use the Basic SSAI Player sample as a starting point for your code.
- In the ViewController.swift file, replace the following with your own values:
- Account Id
- Video Id
- Policy Key
-
Replace the
AdConfigId
value with your playback token.static let AdConfigId = "your playback token"
-
The
AdConfigId
with your playback token value is added as a query parameter.let queryParameters = [kBCOVPlaybackServiceParamaterKeyAdConfigId: Constants.AdConfigId]
-
Make the catalog call to the Playback API with your live stream video id and the query parameter.
playbackService.findVideo(withVideoID: Constants.VideoId, parameters: queryParameters) { [weak self] (video: BCOVVideo?, jsonResponse: [AnyHashable: Any]?, error: Error?) -> Void in guard let _video = video else { print("ViewController Debug - Error retrieving video: \(error?.localizedDescription ?? "unknown error")") return } self?.playbackController?.setVideos([_video] as NSFastEnumeration) }
- Your player is ready to play your live stream.